Registration Form in Android with Validation
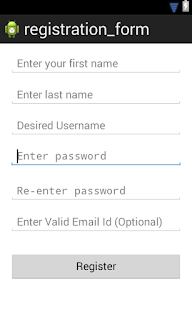
Step 1:
The very first step in any android development or any kind of development is to make an Interface for the user of that application. So we are also doing the same. Firstly we will make an interface for registration form. Copy and paste the following code in res/layout/activity_main.xml file.
activity_main.xml
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<EditText
android:id="@+id/edtUsername"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/edtlastname"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
android:ems="10"
android:hint="@string/desired_username"
android:inputType="textPersonName"
android:singleLine="true" />
<EditText
android:id="@+id/edtfirstname"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtlastname"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/enter_your_first_name"
android:inputType="textPersonName"
android:singleLine="true" />
<EditText
android:id="@+id/edtPass"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtUsername"
android:layout_below="@+id/edtUsername"
android:layout_marginTop="14dp"
android:ems="10"
android:hint="@string/enter_password"
android:inputType="textPassword"
android:singleLine="true" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/edtConfirmPass"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtPass"
android:layout_below="@+id/edtPass"
android:layout_marginTop="21dp"
android:ems="10"
android:hint="@string/re_enter_password"
android:inputType="textPassword"
android:singleLine="true" />
<EditText
android:id="@+id/edtEmail"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtConfirmPass"
android:layout_below="@+id/edtConfirmPass"
android:layout_marginTop="16dp"
android:ems="10"
android:hint="@string/enter_valid_email_id_optional_"
android:inputType="textEmailAddress"
android:singleLine="true" />
<EditText
android:id="@+id/edtlastname"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtUsername"
android:layout_below="@+id/edtfirstname"
android:layout_marginTop="19dp"
android:ems="10"
android:hint="@string/enter_last_name"
android:inputType="textPersonName"
android:singleLine="true" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/edtEmail"
android:layout_below="@+id/edtEmail"
android:layout_marginTop="35dp"
android:text="@string/register" />
</RelativeLayout>
</ScrollView>
|
Step 2:
Now your interface for registration form is ready. Now all you have to do is apply some functionality on the different controls of registration form. Follow these steps:
· Make objects of EditTexts and Button.
Button btnReg;
EditText edtFirst,edtLast, edtUser, edtPass, edtConfPass, edtEmail;
|
· Initialize all the controls.
edtFirst=(EditText)findViewById(R.id.edtfirstname);
edtLast=(EditText)findViewById(R.id.edtlastname);
edtUser=(EditText)findViewById(R.id.edtUsername);
edtPass=(EditText)findViewById(R.id.edtPass);
edtConfPass=(EditText)findViewById(R.id.edtConfirmPass);
edtEmail=(EditText)findViewById(R.id.edtEmail);
//Initialization of Register Button
btnReg=(Button)findViewById(R.id.button1);
|
· Now apply click listener to the Button.
//Registration button functionality
btnReg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
if(edtFirst.getText().toString().length()==0){
edtFirst.setError("First name not entered");
edtFirst.requestFocus();
}
if(edtLast.getText().toString().length()==0){
edtLast.setError("Last name not entered");
edtLast.requestFocus();
}
if(edtUser.getText().toString().length()==0){
edtUser.setError("Username is Required");
edtUser.requestFocus();
}
if(edtPass.getText().toString().length()==0){
edtPass.setError("Password not entered");
edtPass.requestFocus();
}
if(edtConfPass.getText().toString().length()==0){
edtConfPass.setError("Please confirm password");
edtLast.requestFocus();
}
if(!edtPass.getText().toString().equals(edtConfPass.getText().toString())){
edtConfPass.setError("Password Not matched");
edtConfPass.requestFocus();
}
if(edtPass.getText().toString().length()<8 span="">
edtPass.setError("Password should be atleast of 8 charactors");
edtPass.requestFocus();
}
else {
Toast.makeText(getApplicationContext(),"Success", Toast.LENGTH_LONG).show();
}
}
});
|
Your Final Code in MainActivity.java file will be:
MainActivity.java
package com.AT4U.registration_form;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
Button btnReg;
EditText edtFirst,edtLast, edtUser, edtPass, edtConfPass, edtEmail;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//initialization of all editText
edtFirst=(EditText)findViewById(R.id.edtfirstname);
edtLast=(EditText)findViewById(R.id.edtlastname);
edtUser=(EditText)findViewById(R.id.edtUsername);
edtPass=(EditText)findViewById(R.id.edtPass);
edtConfPass=(EditText)findViewById(R.id.edtConfirmPass);
edtEmail=(EditText)findViewById(R.id.edtEmail);
//Initialization of Register Button
btnReg=(Button)findViewById(R.id.button1);
//Registration button functionality
btnReg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
if(edtFirst.getText().toString().length()==0){
edtFirst.setError("First name not entered");
edtFirst.requestFocus();
}
if(edtLast.getText().toString().length()==0){
edtLast.setError("Last name not entered");
edtLast.requestFocus();
}
if(edtUser.getText().toString().length()==0){
edtUser.setError("Username is Required");
edtUser.requestFocus();
}
if(edtPass.getText().toString().length()==0){
edtPass.setError("Password not entered");
edtPass.requestFocus();
}
if(edtConfPass.getText().toString().length()==0){
edtConfPass.setError("Please confirm password");
edtLast.requestFocus();
}
if(!edtPass.getText().toString().equals(edtConfPass.getText().toString())){
edtConfPass.setError("Password Not matched");
edtConfPass.requestFocus();
}
if(edtPass.getText().toString().length()<8 span="">
edtPass.setError("Password should be atleast of 8 charactors");
edtPass.requestFocus();
}
else {
Toast.makeText(getApplicationContext(),"Success", Toast.LENGTH_LONG).show();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
|
Registration Form in Android with Validation
Reviewed by Anonymous
on
February 26, 2017
Rating:

No comments: